The good news is that indentation errors in python are some of the easiest issues to fix. Mastering proper indentation not only resolves these errors but also significantly improves your Python coding skills. In this blog post, we’ll explore the common causes of indentation errors, practical solutions to address them, and tips for writing clean, professional Python code that eliminates such mistakes entirely
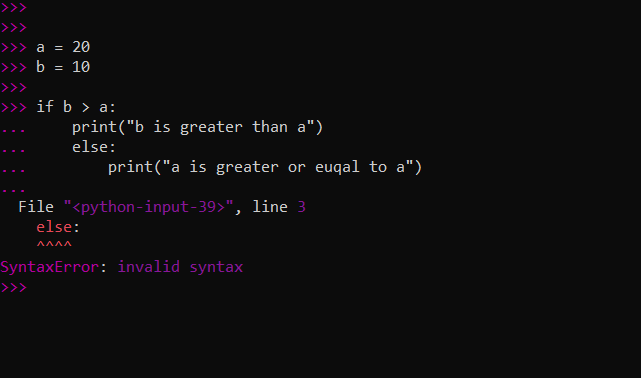
What Are Indentation Errors in Python?
Python uses indentation to define blocks of code. Unlike many other programming languages that use braces or keywords, Python relies on whitespace. This makes Python code more readable but also means that inconsistent indentation can lead to errors.
A common error message looks like this:
>>> a = 100
>>> b = 200
>>>
>>> if a > b:
... print("a is larger than b")
... else:
... elif a < b:
... print("b is larger than a")
... else:
... print("a and b are euqal")
...
File "<python-input-7>", line 2
print("a is larger than b")
^^^^^
IndentationError: expected an indented block after 'if' statement on line 1
>>>
This error occurs because Python expects an indented block of code after control structures like if
, for
, while
, or function definitions.
Common Causes of Indentation Errors in Python
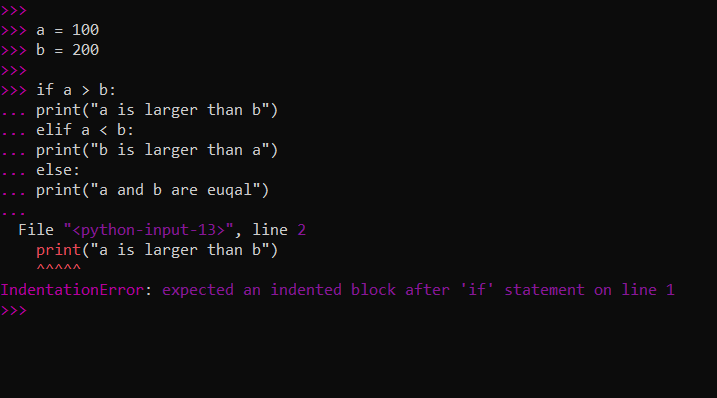
- Unreliable Tab and Space Usage: Python can become confused if tabs and spaces are mixed together in the same file.
- Absence of indentation: failing to use an if statement or other control structure before indenting code.
- Additional Indentation: putting extraneous spaces at the start of a line.
- Pasting Code Copy: Because of formatting changes, code copied from one source to another may not be indented correctly.
- Blocks that are not aligned: Blocks of code that do not follow the intended structure.
How to Fix Indentation Errors in Python
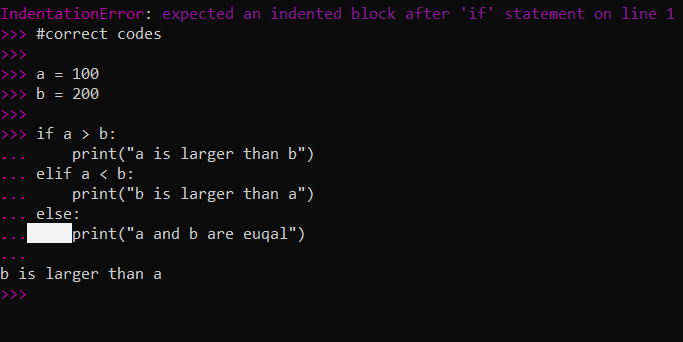
Step 1: Determine the Mistake
- Pay close attention to the error message; it indicates the location of the issue.
- Examine the specified file and line number.
Step 2: Verify your indentation
- Make sure your code is consistently indented.
- Four spaces are advised for each indentation level in Python.
- Don’t mix spaces and tabs. Make consistent use of spaces.
Step 3: Turn on Editor Functionalities
- Make use of an IDE or text editor that supports Python, like Sublime Text, PyCharm, or Visual Studio Code.
- In order to see tabs and spaces, turn on “Show Whitespace Characters”.
- Turn on “Auto-Indent” to keep your indentation constant.
Step 4: Correct the indentation.
- Logically align code chunks to fix the indentation.
- Make sure the indentation of each line in a block is the same.
Step 5: Make Use of a Linter
- Pylint or flake8 liners can detect and correct indentation problems.
- To obtain comprehensive recommendations, install and run the linter.
Correct codes of above indentation error:
>>> #correct codes
>>>
>>> a = 100
>>> b = 200
>>>
>>> if a > b:
print("a is larger than b")
elif a < b:
print("b is larger than a")
else:
print("a and b are euqal")
output:
a is greater or euqal to b
Best Practices for Avoiding Indentation Errors in Python
- Adopt a Consistent Style: Observe the PEP 8 indentation guidelines.
- Employ a Code Editor: Indentation can be automatically handled and problems can be detected by modern editors.
- Make use of version control: Git and other similar tools can be used to track changes and swiftly find problematic code.
- Write code that is modular: Break your code into smaller functions to make it easier to handle and easy to manage.
- Learn Python Syntax Properly: Take the time to learn Python’s code structure and indentation guidelines.
Conclusion
Indentation errors may seem daunting at first, but they are easy to fix with the right approach. By using consistent indentation, leveraging the tools available in modern IDEs, and following Python’s an extra practice , you can avoid these errors entirely and write cleaner, more professional code. Remember, mastering indentation is not just about fixing errors; it’s about making your code more readable and maintainable. Happy coding!
Thanks 👍
Pingback: how do i turn the ai off on my gmail - Learn to Code and Solve Errors with Walchats