1. Introduction
Understanding loop control in Python (break, continue, pass) is essential for efficiently managing loops. These statements help programmers control the flow of loops, allowing them to exit, skip, or ignore specific iterations as needed.
In this guide, we will explore how to use break
, continue
, and pass
in Python, real-life applications, common mistakes, and how to fix them.
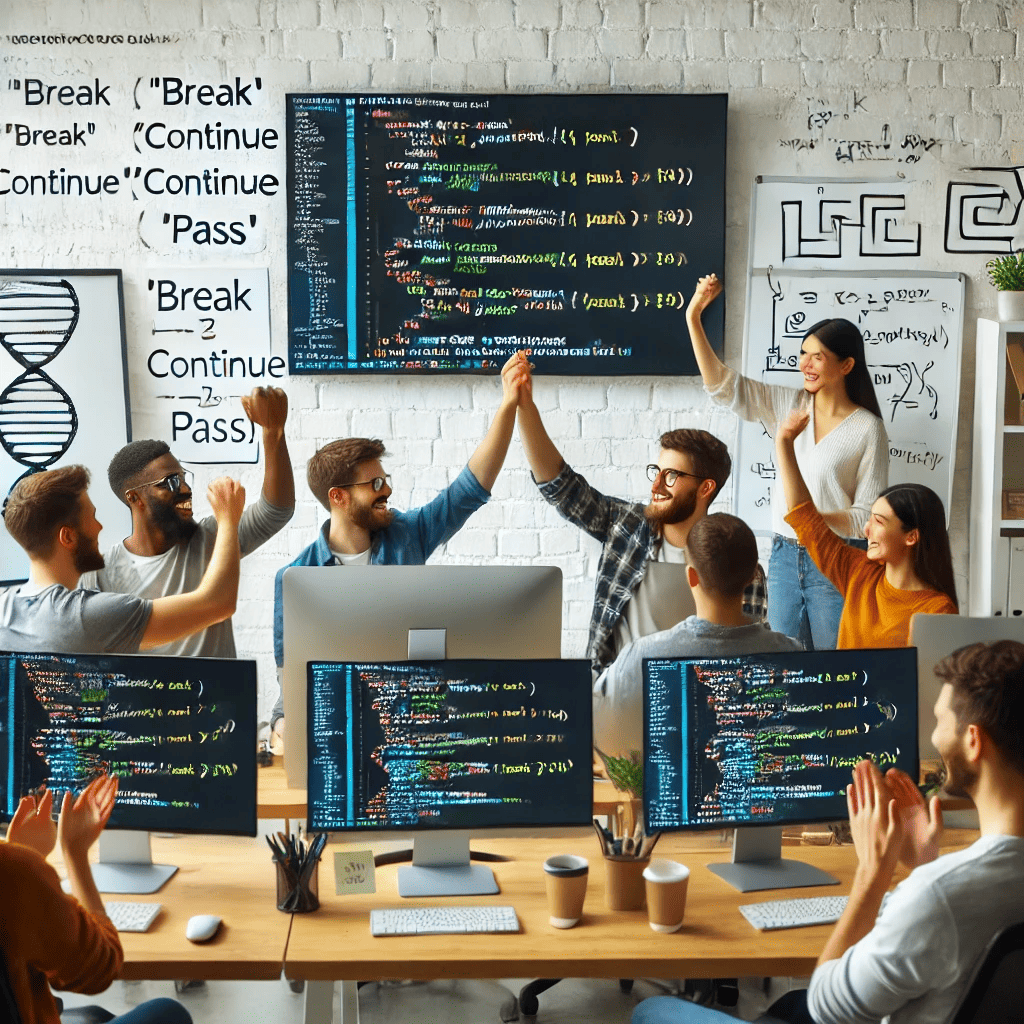
Focus Keyphrase: Loop Control in Python (break, continue, pass)
2. What is Loop Control in Python?
Loop control statements modify the execution of loops:
break
– Exits the loop immediately.continue
– Skips the current iteration and moves to the next.pass
– Does nothing but acts as a placeholder for future code.
3. Using break
, continue
, and pass
in Python
a) break
Statement
The break
statement terminates the loop when a condition is met.
for num in range(10):
if num == 5:
break # Loop stops when num reaches 5
print(num)
Output:
0
1
2
3
4
b) continue
Statement
The continue
statement skips the current iteration and moves to the next.
for num in range(5):
if num == 3:
continue # Skips number 3
print(num)
Output:
0
1
2
4
c) pass
Statement
The pass
statement does nothing but acts as a placeholder for future code.
for num in range(5):
if num == 3:
pass # Placeholder; loop continues as normal
print(num)
Output:
0
1
2
3
4
4. Real-Life Applications of Loop Control Statements
a) User Authentication System (break
)
while True:
password = input("Enter password: ")
if password == "secure123":
print("Access granted")
break # Exits loop when correct password is entered
print("Wrong password. Try again.")
b) Skipping Invalid User Input (continue
)
ages = [25, -5, 30, -2, 40] # Negative values are invalid
for age in ages:
if age < 0:
continue # Skip negative values
print(f"Valid age: {age}")
Output:
Valid age: 25
Valid age: 30
Valid age: 40
c) Placeholder for Future Code (pass
)
def process_data(data):
if data == "":
pass # To be implemented later
else:
print(f"Processing {data}")
process_data("Sample Data")
Output:
Processing Sample Data
5. Common Mistakes and How to Fix Them
Mistake 1: Using break
Incorrectly in for
Loops
❌ Incorrect:
for i in range(5):
print(i)
break # Ends loop immediately, printing only 0
✅ Fix: Use break
only when a specific condition is met.
for i in range(5):
if i == 3:
break
print(i)
Output:
0
1
2
Mistake 2: Forgetting continue
Skips Important Code
❌ Incorrect:
for i in range(5):
if i == 2:
continue
print("Processing", i) # Skips processing 2
✅ Fix: Ensure skipping an iteration doesn’t break program logic.
Mistake 3: Using pass
Instead of continue
❌ Incorrect:
for i in range(5):
if i == 3:
pass # Does nothing but doesn't skip iteration
print(i)
✅ Fix: Use continue
to actually skip.
for i in range(5):
if i == 3:
continue # Skips 3
print(i)
6. Conclusion
Mastering loop control in Python (break, continue, pass) helps improve efficiency and readability in loops. The break
statement stops loops, continue
skips iterations, and pass
acts as a placeholder for future code.
Next Steps:
- Experiment with
break
,continue
, andpass
in different scenarios. - Ensure
continue
andbreak
do not disrupt program logic. - Use
pass
when defining functions or loops for future implementation.