1. Introduction
Understanding loops in Python (for, while) is essential for automating repetitive tasks. Loops allow us to execute a block of code multiple times, making programs efficient and reducing redundancy.
In this guide, we will explore how to use for
and while
loops in Python, real-life applications, common mistakes, and how to fix them.
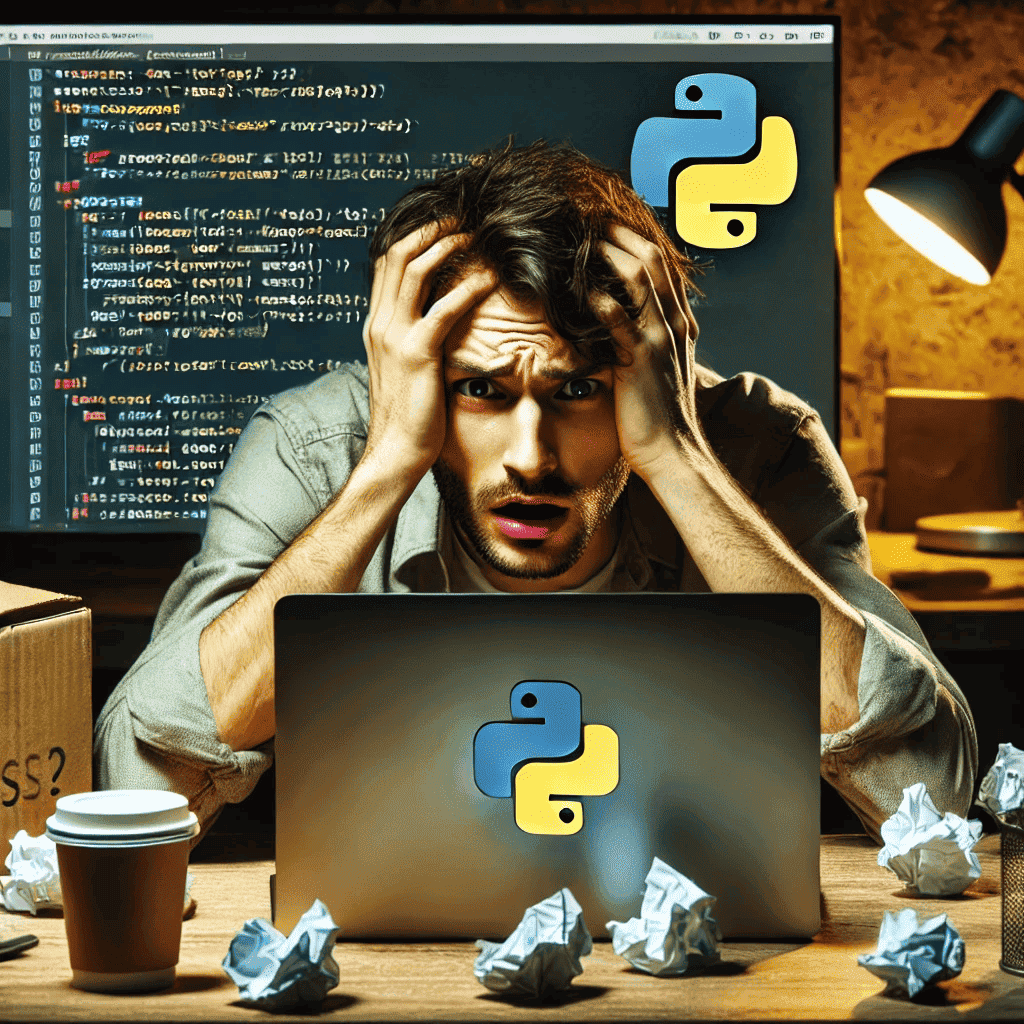
Focus Keyphrase: Loops in Python (for, while)
2. What are Loops in Python?
Loops are control structures that repeat a block of code as long as a condition is met. Python provides two main types of loops:
for
Loop – Iterates over a sequence (e.g., list, tuple, string).while
Loop – Repeats as long as a condition remains true.
3. Using for
and while
Loops in Python
a) for
Loop
A for
loop is used when we know how many times we want to repeat an action.
for i in range(5): # Loops from 0 to 4
print("Iteration:", i)
Output:
Iteration: 0
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
b) Iterating Over a List with for
Loop
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
c) while
Loop
A while
loop runs as long as the condition is true.
count = 0
while count < 3:
print("Count:", count)
count += 1
Output:
Count: 0
Count: 1
Count: 2
d) Using break
and continue
break
– Stops the loop immediately.continue
– Skips the current iteration and moves to the next.
for num in range(5):
if num == 3:
break # Stops loop when num is 3
print(num)
Output:
0
1
2
for num in range(5):
if num == 3:
continue # Skips 3
print(num)
Output:
0
1
2
4
4. Real-Life Applications of Loops
a) Automating Repetitive Tasks
for i in range(10):
print(f"Processing item {i}...")
b) User Login System with Retry Attempts
attempts = 3
while attempts > 0:
password = input("Enter password: ")
if password == "secret":
print("Access granted")
break
else:
attempts -= 1
print(f"Wrong password. {attempts} attempts left.")
c) Web Scraping (Extracting Data from Websites)
import requests
urls = ["https://example.com", "https://another.com"]
for url in urls:
response = requests.get(url)
print(f"Fetched {url} - Status Code: {response.status_code}")
5. Common Mistakes and How to Fix Them
Mistake 1: Forgetting to Update the Condition in while
Loop
❌ Incorrect:
count = 0
while count < 5:
print("Infinite loop!") # The loop runs forever because count is not updated
✅ Fix: Update the condition inside the loop.
count = 0
while count < 5:
print("Count:", count)
count += 1
Mistake 2: Off-by-One Errors in for
Loop
❌ Incorrect:
for i in range(1, 5): # Expected to loop 5 times but only loops 4 times
print(i)
✅ Fix: Adjust the range to include the correct number of iterations.
for i in range(1, 6):
print(i) # Loops exactly 5 times
Mistake 3: Using while True
Without a break
Statement
❌ Incorrect:
while True:
print("This loop will never stop!") # Runs indefinitely
✅ Fix: Use break
to exit the loop when a condition is met.
while True:
response = input("Enter 'exit' to stop: ")
if response == "exit":
break
Mistake 4: Using for
When while
is Needed
❌ Incorrect:
for i in range(5):
if i == 3:
break
print(i)
✅ Fix: Use while
if the number of iterations is unknown.
count = 0
while count < 5:
print(count)
if count == 3:
break
count += 1
6. Conclusion
Mastering loops in Python (for, while) is crucial for automating tasks, iterating through data, and optimizing code efficiency. Use for
loops when the number of iterations is known and while
loops when conditions determine the repetition.
Next Steps:
- Practice using loops in different scenarios.
- Experiment with
break
andcontinue
statements. - Avoid common mistakes like infinite loops and incorrect ranges.