Type Conversion in Python (int, float, str)
1. Introduction
Understanding type conversion in Python (int, float, str) is essential for handling user input, performing calculations, and managing data efficiently. Python provides built-in functions like int()
, float()
, and str()
to convert values between different data types.
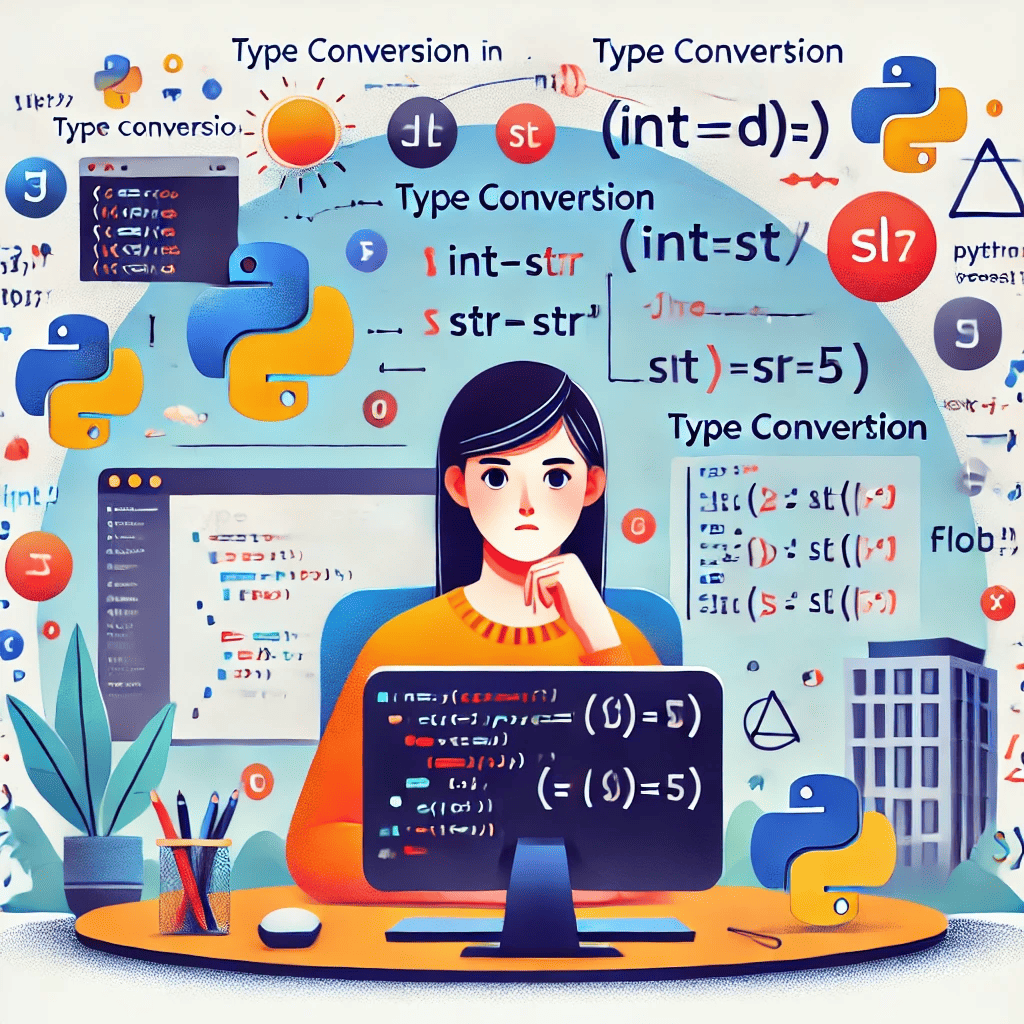
In this guide, we will explore type conversion methods, real-life applications, common mistakes, and how to fix them.
Focus Keyphrase: Type Conversion in Python (int, float, str)
2. What is Type Conversion?
Type conversion is the process of changing a variable’s data type. There are two types:
- Implicit Conversion: Done automatically by Python.
- Explicit Conversion (Type Casting): Done manually using functions like
int()
,float()
, andstr()
.
3. Implicit Type Conversion (Automatic by Python)
Python automatically converts smaller data types to larger ones when necessary.
Example of Implicit Conversion
num_int = 5
num_float = num_int + 2.5 # int is converted to float automatically
print(num_float)
print(type(num_float))
Output:
7.5
<class 'float'>
Why does this happen?
Python converts int
to float
to avoid data loss in calculations.
4. Explicit Type Conversion (Using int(), float(), str())
a) Converting to Integer (int()
)
Used when you need whole numbers.
num_float = 3.8
num_int = int(num_float) # Converts float to int (removes decimal part)
print(num_int)
Output:
3
⚠️ Note: int()
does not round the number; it only removes the decimal part.
Real-Life Application:
- Age verification: Converting user input (string) to an integer.
age = int(input("Enter your age: ")) # User enters "25" (string), converted to int
if age >= 18:
print("You are an adult.")
b) Converting to Float (float()
)
Used when you need decimal numbers.
num_int = 5
num_float = float(num_int) # Converts int to float
print(num_float)
Output:
5.0
Real-Life Application:
- E-commerce calculations: Converting product price from string to float for accurate billing.
price = float(input("Enter product price: ")) # User enters "49.99"
print(f"Total after tax: {price * 1.15}") # Applying 15% tax
c) Converting to String (str()
)
Used when you need to concatenate numbers with text.
num = 100
num_str = str(num) # Converts int to string
print("The total amount is " + num_str)
Output:
The total amount is 100
Real-Life Application:
- Displaying messages dynamically:
name = "John"
balance = 500
print("Hello " + name + ", your balance is " + str(balance))
5. Common Mistakes and How to Fix Them
Mistake 1: Forgetting to Convert Input to Integer or Float
❌ Incorrect:
age = input("Enter your age: ") # Input is always a string
if age >= 18: # TypeError: '>' not supported between str and int
print("You are an adult.")
✅ Fix: Convert input to int()
.
age = int(input("Enter your age: "))
if age >= 18:
print("You are an adult.")
Mistake 2: Using int()
on Non-Numeric Strings
❌ Incorrect:
num = int("hello") # ValueError: invalid literal for int()
✅ Fix: Always check if the input is numeric before conversion.
user_input = input("Enter a number: ")
if user_input.isdigit():
num = int(user_input)
print("Valid number:", num)
else:
print("Invalid input! Please enter a numeric value.")
Mistake 3: Expecting int()
to Round Numbers
❌ Incorrect:
num = int(4.9) # Expecting 5, but gets 4
✅ Fix: Use round()
before converting.
num = round(4.9) # Rounds to 5 before converting
print(num) # Output: 5
6. Conclusion
Mastering type conversion in Python (int, float, str) helps in handling user input, performing calculations, and formatting data correctly. Python supports both implicit conversion (automatic) and explicit conversion (using functions like int()
, float()
, and str()
).
Next Steps:
- Practice converting different data types.
- Handle user input correctly to avoid errors.
- Use type conversion in real-life applications like billing systems and login forms.