1. Introduction
Understanding Python syntax and indentation is essential for writing clean and error-free code. Unlike other programming languages that use curly brackets {}
to define blocks of code, Python relies on indentation to structure programs properly. Incorrect indentation can lead to errors, making it crucial for beginners to grasp these concepts early.
In this guide, we will explore Python syntax, indentation rules, examples, real-life applications, common mistakes, and how to fix them.
Focus Keyphrase: Understanding Python Syntax and Indentation
2. What is Python Syntax?
Python syntax refers to the set of rules that dictate how Python code should be written and formatted. Here are a few key points:
- Python is case-sensitive (
print
andPrint
are different). - Each statement is written on a new line (unless using semicolons
;
). - Indentation is mandatory (used to define blocks of code).
- Python uses colons (
:
) to start code blocks (e.g., loops, functions).
Example of Python Syntax
# This is a comment
name = "Alice" # Variable assignment
print("Hello, " + name) # Output: Hello, Alice
3. What is Python Indentation?
Indentation in Python refers to spaces or tabs used at the beginning of a line to define code blocks. Python enforces indentation to improve code readability and structure.
Example of Correct Indentation
def greet():
print("Hello, World!") # Indented correctly
greet() # Function call
Incorrect Indentation (Causes an Error)
def greet():
print("Hello, World!") # No indentation - will cause an error
greet()
Error:
IndentationError: expected an indented block
4. Python Indentation Rules
- Use four spaces per indentation level (recommended).
- The same indentation level should be used within a block.
- Mixing tabs and spaces is not allowed.
- Indentation is required for loops, functions, and conditionals.
Example of Indentation in Loops and Conditionals
age = 18
if age >= 18:
print("You are an adult.") # Indented block
else:
print("You are a minor.")
Example of Indentation in Loops
for i in range(3):
print("Iteration:", i) # Inside loop
print("Loop finished") # Outside loop (no indentation)
5. Real-Life Applications of Python Indentation
Python’s indentation improves readability, making it widely used in:
a) Web Development (Django, Flask)
Example: Django uses indentation to define views and models.
def home(request):
return HttpResponse("Welcome to my website!")
b) Data Science & Machine Learning (Pandas, NumPy)
Example: Analyzing data with Pandas requires properly indented loops.
import pandas as pd
data = {"Name": ["Alice", "Bob"], "Age": [25, 30]}
df = pd.DataFrame(data)
for index, row in df.iterrows():
print(row["Name"], "is", row["Age"], "years old")
c) Automation & Scripting
Example: A script to rename files using indentation.
import os
for file in os.listdir():
if file.endswith(".txt"):
print("Processing file:", file)
6. Common Mistakes and How to Fix Them
Mistake 1: Mixing Tabs and Spaces in python syntax
❌ Incorrect:
def hello():
tabbed = "This uses tabs"
spaced = "This uses spaces"
✅ Fix: Use only spaces for indentation.
Mistake 2: Inconsistent Indentation
❌ Incorrect:
def example():
print("Line 1")
print("Line 2") # Extra indentation
✅ Fix: Ensure consistent indentation.
def example():
print("Line 1")
print("Line 2")
Mistake 3: Forgetting to Indent Inside Loops or Conditionals
❌ Incorrect:
for i in range(3):
print(i) # No indentation (causes an error)
✅ Fix: Indent the print statement inside the loop.
for i in range(3):
print(i)
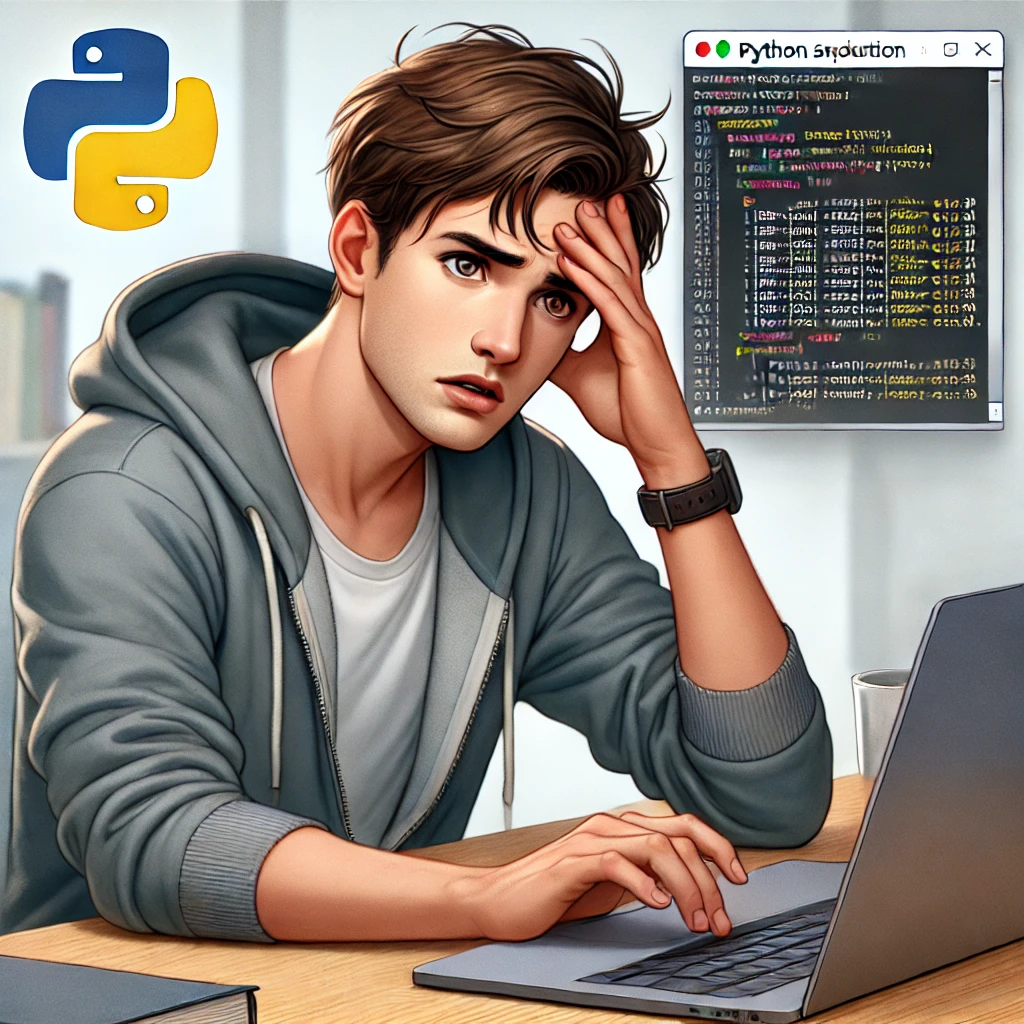
7. Conclusion
Understanding Python syntax and indentation is crucial for writing clean and error-free code. Python enforces indentation to improve readability and maintainability. By following proper indentation rules, beginners can avoid errors and write structured programs efficiently.
Next Steps:
- Practice writing Python programs with correct indentation.
- Use an IDE like VS Code or PyCharm, which highlights indentation errors.
- Explore Python’s loops, functions, and conditionals to improve coding skills.