Variables and Data Types in Python
1. Introduction
Variables and data types are essential in Python. Variables store values, while data types define the kind of data stored. Python has data types like integers, floats, strings, booleans, lists, tuples, dictionaries, and sets.
In this guide, we will explore variables, data types, examples, real-life applications, common mistakes, and how to fix them.
2. What are Variables in Python?
A variable is a name that stores data in memory. In Python, you don’t need to declare variable types explicitly.
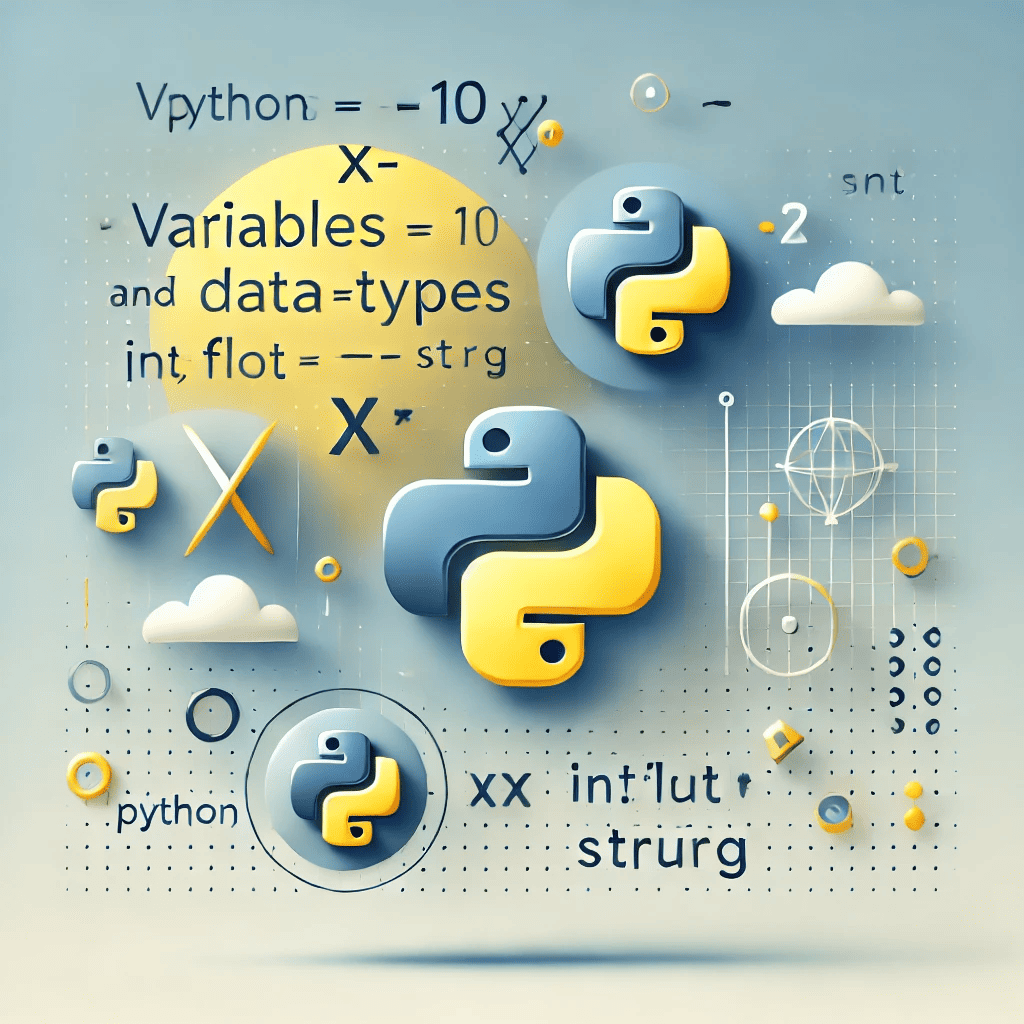
Example of Variables
name = "Alice" # String
age = 25 # Integer
height = 5.7 # Float
is_student = True # Boolean
print(name, age, height, is_student)
Output:
Alice 25 5.7 True
3. Understanding Data Types in Python
Python has several built-in data types, including:
Data Type | Example | Description |
---|---|---|
Integer (int ) | age = 30 | Whole numbers |
Float (float ) | pi = 3.14 | Decimal numbers |
String (str ) | name = "John" | Text data |
Boolean (bool ) | is_raining = False | True or False values |
List (list ) | fruits = ["apple", "banana"] | Ordered, changeable collection |
Tuple (tuple ) | coordinates = (10, 20) | Ordered, unchangeable collection |
Dictionary (dict ) | person = {"name": "Alice", "age": 25} | Key-value pairs |
Set (set ) | unique_numbers = {1, 2, 3} | Unordered collection of unique values |
4. Examples of Variables and Data Types
Example 1: Using Different Data Types
name = "Bob"
age = 30
salary = 5000.50
is_employee = True
print(f"Employee: {name}, Age: {age}, Salary: {salary}, Status: {is_employee}")
Example 2: Lists and Dictionaries in Real-Life Applications
Scenario: A shopping cart in an e-commerce website.
cart = ["Laptop", "Mouse", "Keyboard"]
cart_prices = {"Laptop": 1000, "Mouse": 20, "Keyboard": 50}
print(f"Cart Items: {cart}")
print(f"Price of Laptop: ${cart_prices['Laptop']}")
5. Real-Life Applications of Variables and Data Types
Python variables and data types are used in various applications, including:
a) Web Development
- Storing user information (
name
,email
,password
). - Managing session data using dictionaries.
b) Data Science & Machine Learning
- Lists and NumPy arrays to store datasets.
- Floats and integers for statistical calculations.
c) Finance & Banking
- Using floats for transactions and balances.
- Dictionaries for storing customer account details.
6. Common Mistakes and How to Fix Them
Mistake 1: Using the Wrong Data Type
❌ Incorrect: Trying to add a string to an integer.
age = "25" # String
new_age = age + 1 # Error: Cannot add string and integer
✅ Fix: Convert the string to an integer first.
age = "25"
new_age = int(age) + 1
print(new_age) # Output: 26
Mistake 2: Using an Undefined Variable
❌ Incorrect:
print(score) # Error: score is not defined
✅ Fix: Define the variable before using it.
score = 100
print(score) # Output: 100
Mistake 3: Modifying a Tuple (Immutable Type)
❌ Incorrect:
numbers = (1, 2, 3)
numbers[0] = 10 # Error: Cannot modify a tuple
✅ Fix: Use a list instead of a tuple if modification is needed.
numbers = [1, 2, 3]
numbers[0] = 10
print(numbers) # Output: [10, 2, 3]
7. Conclusion
Variables and data types in Python are the building blocks of programming. They help store, process, and manipulate data efficiently. By understanding the correct usage, beginners can avoid common errors and write better programs. Explore more
Next Steps:
- Experiment with different data types in Python.
- Practice creating variables and performing operations.
- Learn about advanced data structures like lists, dictionaries, and sets.